Finding Area
Let's use a Monte-Carlo type method to calculate area. Suppose we have the following circular dart board and want to know its area.
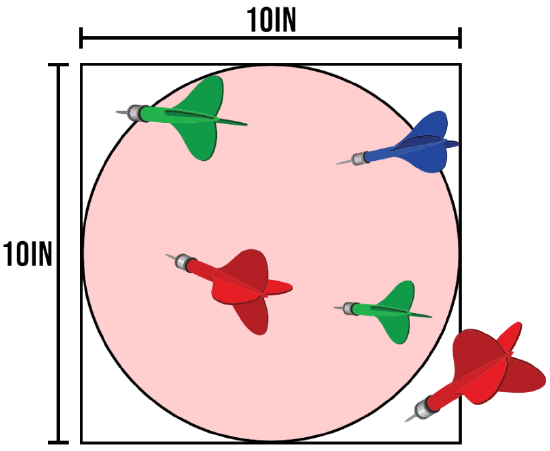
We enclose the dart board with a square of known area, in this case 100in2, and perform following the following procedure:
- Throw darts at the square, being careful not to favor any particular spot.
- Record the percentage of darts that land inside the circle.
- Multiply this percentage by the known area of the square (100in2)
- That's our approximation!
Of course, doing this by hand would take a lot of time. Instead, we can use Python. In our program, we will randomly choose an x-value and y-value using the random.uniform(start,stop) function. This function returns a random float in [start,stop). We will center our circle at the origin, so we will use random.uniform(-5,5) for both the x and y values.
Next, we need to have a way to determine whether the point (x,y) lies inside the circle or not. We know that the equation of the circle under discussion will be
x2+y2=52
Solving for y, we get
y=±√25−x2.
Thus, in our python code, we will check that
y≤√25−x2 and y≥−√25−x2.
That is, that it is below the top half of the circle and above the bottom half of the circle. Here we go!
05 | for a in range ( 1 ,tries + 1 ): |
06 | x = random.uniform( - 5 , 5 ) |
07 | y = random.uniform( - 5 , 5 ) |
08 | if (y< = ( 25 - x * * 2 ) * * ( 1 / 2 )) and (y> = - ( 25 - x * * 2 ) * * ( 1 / 2 )): |
10 | print ( 100 * success / tries) |
output: 78.52
Above, the percentage of "darts" that hit the circle is given by success/tries. We then multiplied that by the area of the square (100) to get the approximate area of the circle, which is given as 78.52, very close to our expected value of 25π.
For fun, we could plot each of the dots to see what our board looks like. Here is an example with 100 dots:
03 | import matplotlib.pyplot as plt |
05 | xc = np.linspace( - 5 , 5 , 1000 ) |
07 | zc = - ( 25 - xc * * 2 ) * * ( 1 / 2 ) |
08 | plt.plot(xc, yc, color = 'red' , linewidth = 2.0 , linestyle = '-' ) |
09 | plt.plot(xc, zc, color = 'red' , linewidth = 2.0 , linestyle = '-' ) |
14 | ax.set_aspect( 'equal' , adjustable = 'box' ) |
18 | for a in range ( 1 ,tries + 1 ): |
19 | x = random.uniform( - 5 , 5 ) |
20 | y = random.uniform( - 5 , 5 ) |
21 | if (y< = ( 25 - x * * 2 ) * * ( 1 / 2 )) and (y> = - ( 25 - x * * 2 ) * * ( 1 / 2 )): |
23 | plt.plot(x,y, 'o' ,color = 'black' ) |
24 | print ( 100 * success / tries) |
output: 80

Integrals
Now that we've found area using a Monte-Carlo type simulation, you might have an idea of how to calculate integrals: just find the area under the curve!
Example: Use a Monte-Carlo type simulation to find the area under the curve over [0,4]
y=0.5x2
Answer: To start, we need to find a box that will fit the entire curve we are calculating. The x-values are easy: just use the given interval [0,4]. For the y-values, we need to find the least and greatest y-values that this curve achieves. Since this is a parabola, we can see that the least y-value is 0 and the greatest is 0.5∗(4)2=8. So our box will be [0,4]x[0,8]. That means our random points will have x-values in [0,4] and y-values in [0,8]. We will determine whether the point is above or below the curve similar to the circle example above. We will consider a "success" - that is, consider our point to be under the curve - if:
y≤0.5x2.
Note that we don't need to determine whether the point is above the x-axis because our y-values are only chosen from [0,8]. The area of our box is 4*8=32. Let's modify the above program:
03 | import matplotlib.pyplot as plt |
05 | xc = np.linspace( 0 , 4 , 1000 ) |
07 | plt.plot(xc, yc, color = 'red' , linewidth = 2.0 , linestyle = '-' ) |
11 | for a in range ( 1 ,tries + 1 ): |
16 | plt.plot(x,y, 'o' ,color = 'black' ) |
17 | print ( 32 * success / tries) |
output: 10.208

This is relatively close to our expected value (using integration) of 10.6667. If we increase the "tries" variable, we can get even closer. However, this will make our picture look like a blob!
Exercise
Use the techniques above to approximate the integral ∫ππ/2
- 11
- 3
- −4
- Answer
-
- 111
- 31
- −41